What are Events?
All the different visitor's action that a web page can respond to are a called event.
What is AJAX?
AJAX = Asynchronous JavaScript and XML.
In short; AJAX is about loading data in the background and display it on the webpage, without reloading the whole page.
examples of applications using AJAX: Gmail, Google Maps, Youtube, and Facebook tabs.
you can learn more about AJAX in our AJAX tutorial.
Sunday, May 20, 2018
jQuery Chaining
With jQuery, you can chain together actions/methods
Chaining allow us to run multiple jQuery methods (on the same element) within a single statement.
jQuery Method Chaining
Until now we have been writing jQuery statement one at a time( one after the other)
However, there is a technique called chaining, that allows us to run multiple jQuery commands, one after the other, one the same element(s).
jQuery DOM Manipulation
One very import part of jQuery is the possibility to manipulate the DOM.
jQueyr comes with a bunch of DOM related methods that make it easy to access and manipulate elemens and attributes.
Chaining allow us to run multiple jQuery methods (on the same element) within a single statement.
jQuery Method Chaining
Until now we have been writing jQuery statement one at a time( one after the other)
However, there is a technique called chaining, that allows us to run multiple jQuery commands, one after the other, one the same element(s).
jQuery DOM Manipulation
One very import part of jQuery is the possibility to manipulate the DOM.
jQueyr comes with a bunch of DOM related methods that make it easy to access and manipulate elemens and attributes.
jQuery Dimensions
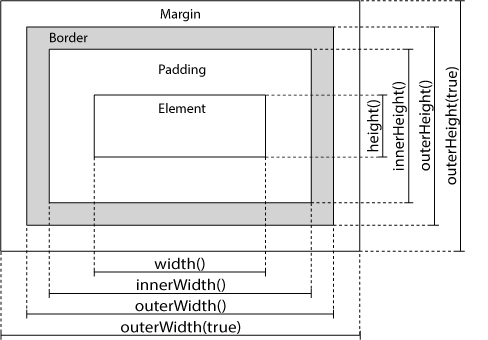
Saturday, May 19, 2018
JQuery
Basic syntax is :$(selector).action()
- A $ sign to define/access JQuery
- A(selector) to "query (or find)" HTML elements
- A jQuery action() to be performed on the elements(s)
examples:
$(this).hide() - hides the current element.
$("p').hide() - hides all <p> elements
$(".test").hide() hides all elements with class ="test"
$("#test").hid() hides all elements with id = "test"
Are you familiar with CSS selector?
jQuery uses CSS syntax to select elements. You will learn more about he selector syntax in the next chapter of this tutorial.
The Document Ready Event
$(document).ready(function ()
{
}
This is to prevent any jQuery code from running before the document is finished loading (is ready).
- A $ sign to define/access JQuery
- A(selector) to "query (or find)" HTML elements
- A jQuery action() to be performed on the elements(s)
examples:
$(this).hide() - hides the current element.
$("p').hide() - hides all <p> elements
$(".test").hide() hides all elements with class ="test"
$("#test").hid() hides all elements with id = "test"
Are you familiar with CSS selector?
jQuery uses CSS syntax to select elements. You will learn more about he selector syntax in the next chapter of this tutorial.
The Document Ready Event
$(document).ready(function ()
{
}
This is to prevent any jQuery code from running before the document is finished loading (is ready).
More Examples of jQuery Selectors
Syntax | Description | Example |
---|---|---|
$("*") | Selects all elements | Try it |
$(this) | Selects the current HTML element | Try it |
$("p.intro") | Selects all <p> elements with class="intro" | Try it |
$("p:first") | Selects the first <p> element | Try it |
$("ul li:first") | Selects the first <li> element of the first <ul> | Try it |
$("ul li:first-child") | Selects the first <li> element of every <ul> | Try it |
$("[href]") | Selects all elements with an href attribute | Try it |
$("a[target='_blank']") | Selects all <a> elements with a target attribute value equal to "_blank" | Try it |
$("a[target!='_blank']") | Selects all <a> elements with a target attribute value NOT equal to "_blank" | Try it |
$(":button") | Selects all <button> elements and <input> elements of type="button" | Try it |
$("tr:even") | Selects all even <tr> elements | Try it |
$("tr:odd") | Selects all odd <tr> elements | Try it |
Use our jQuery Selector Tester to demonstrate the different selectors.
functions In a Separate File
If your website contains a lot of pages, and you want your jQuery functions to be easy to maintain, you can put your jQury functions in a separate .js file.
When we demonstrate jQuery in this tutorial, the functions are added directly into teh <head> section. However, sometimes it is preferable to place them in a separate file, like (use the src attribute to refer to the .js file).
ASP.NET MVC6 default debugging launch URL
refer to https://stackoverflow.com/questions/30002050/asp-net-mvc-6-default-debugging-launch-url
Q: When using the new Visual Studio 2015 RC ASP.NET 5.0 Web API template the default debugging start up URL is somehow set to
Ans:
here was very little documentation that I could find regarding where this start up URL was declared. There is a brief mention of it in this blog post on MSDN. I eventually stumbled upon it in the
Q: When using the new Visual Studio 2015 RC ASP.NET 5.0 Web API template the default debugging start up URL is somehow set to
api/values
. Where is this default configured and how do I change it?Ans:
here was very little documentation that I could find regarding where this start up URL was declared. There is a brief mention of it in this blog post on MSDN. I eventually stumbled upon it in the
launchSettings.json
file under the Properties
node of the project as shown below:
Here are the contents of this file:
{
"profiles": {
"IIS Express": {
"commandName" : "IISExpress",
"launchBrowser": true,
"launchUrl": "api/values",
"environmentVariables" : {
"ASPNET_ENV": "Development"
}
}
}
}
You can change the
launchURL
to your desired default route.Monday, May 14, 2018
Sunday, May 13, 2018
JavaScript JSON
What is JSON?
- JSON stands for JavaScript Object Notation
- JSON is lightweight data interchange format
- JSON is language independent *
- JSON is "self-describing" and easy to understand
* The JSON syntax is derived from JavaScript object notation syntax, but the JSON format is text only. Code for reading and generating JSON data can be written in any programming language.
JSON Example
This JSON syntax defines an employees object: an array of 3 employee records (objects):JSON Example
{
"employees":[
{"firstName":"John", "lastName":"Doe"},
{"firstName":"Anna", "lastName":"Smith"},
{"firstName":"Peter", "lastName":"Jones"}
]
}
The JSON Format Evaluates to JavaScript Objects
The JSON format is syntactically identical to the code for creating JavaScript objects.Because of this similarity, a JavaScript program can easily convert JSON data into native JavaScript objects.
JSON Syntax Rules
- Data is in name/value pairs
- Data is separated by commas
- Curly braces hold objects
- Square brackets hold arrays
Converting a JSON Text to a JavaScript Object
A common use of JSON is to read data from a web server, and display the data in a web page.
For simplicity, this can be demonstrated using a string as input.
First, create a JavaScript string containing JSON syntax:
var text = '{ "employees" : [' +
'{ "firstName":"John" , "lastName":"Doe" },' +
'{ "firstName":"Anna" , "lastName":"Smith" },' +
'{ "firstName":"Peter" , "lastName":"Jones" } ]}';
Then, use the JavaScript built-in function JSON.parse() to convert the string into a JavaScript object:
var obj = JSON.parse(text);
Finally, use the new JavaScript object in your page:
Example
<p id="demo"></p>
<script>
document.getElementById("demo").innerHTML =
obj.employees[1].firstName + " " + obj.employees[1].lastName;</script>
JS Reserved Words
avaScript Objects, Properties, and Methods
You should also avoid using the name of JavaScript built-in objects, properties, and methods:Array | Date | eval | function |
hasOwnProperty | Infinity | isFinite | isNaN |
isPrototypeOf | length | Math | NaN |
name | Number | Object | prototype |
String | toString | undefined | valueOf |
Java Reserved Words
JavaScript is often used together with Java. You should avoid using some Java objects and properties as JavaScript identifiers:getClass | java | JavaArray | javaClass |
JavaObject | JavaPackage |
Other Reserved Words
JavaScript can be used as the programming language in many applications.
You should also avoid using the name of HTML and Window objects and properties:alert | all | anchor | anchors |
area | assign | blur | button |
checkbox | clearInterval | clearTimeout | clientInformation |
close | closed | confirm | constructor |
crypto | decodeURI | decodeURIComponent | defaultStatus |
document | element | elements | embed |
embeds | encodeURI | encodeURIComponent | escape |
event | fileUpload | focus | form |
forms | frame | innerHeight | innerWidth |
layer | layers | link | location |
mimeTypes | navigate | navigator | frames |
frameRate | hidden | history | image |
images | offscreenBuffering | open | opener |
option | outerHeight | outerWidth | packages |
pageXOffset | pageYOffset | parent | parseFloat |
parseInt | password | pkcs11 | plugin |
prompt | propertyIsEnum | radio | reset |
screenX | screenY | scroll | secure |
select | self | setInterval | setTimeout |
status | submit | taint | text |
textarea | top | unescape | untaint |
window |
HTML Event Handlers
In addition you should avoid using the name of all HTML event handlers.
Examples:onblur | onclick | onerror | onfocus |
onkeydown | onkeypress | onkeyup | onmouseover |
onload | onmouseup | onmousedown | onsubmit |
JavaScript Style Guide and Coding Conventions
Always use the same coding conventions for all your JavaScript projects.
JavaScript Coding Conventions
Coding conventions are style guidelines for programming. They typically cover:
JavaScript Coding Conventions
Coding conventions are style guidelines for programming. They typically cover:
- Naming and declaration rules for variables and functions.
- Rules for the use of white space, indentation, and comments.
- Programming practices and princiles
Coding conventions secure quality:
- Improves code readability
- Make code maintenance easier
Coding conventions can be documented rules for teams to follow, or just be your individual coding practice.
Never Declare Number, String, or Boolean Objects
Always treat numbers, strings, or booleans as primitive values. Not as objects.
Declaring these types as objects, slows down execution speed, and products nasty side effects:
Use === Comparison
The == comparison operator always converts (to matching types) before comparison.
The === operator forces comparison of values and type:
Example
0 == ""; // true1 == "1"; // true1 == true; // true
0 === ""; // false1 === "1"; // false1 === true; // false
Try it Yourself »Use Parameter Defaults
If a function is called with a missing argument, the value of the missing argument is set to undefined.
Undefined values can break your code. It is a good habit to assign default values to arguments.
Read more about function parameters and arguments at Function Parameters
Avoid Using eval()
The eval() function is used to run text as code. In almost all cases, it should not be necessary to use it.
Because it allows arbitrary code to be run, it also represents a security problem.
JavaScript Debugging
❝
Errors can (will) happen, every time you write some new computer code.
JavaScript Debuggers
Debugging is not easy. But fortunately, all modern browsers have a built-in JavaScript debugger.
Built-in debuggers can be turned on and off, forcing errors to be reported to the user.
With a debugger, you can also set breakpoints (places where code execution can be stopped), and examine variables while the code is executing.
Normally, otherwise follow the steps at the bottom of this page, you activate debugging in your browser with the F12 key, and select "Console" in the debugger menu.
The console.log() Method
If your browser supports debugging, you can use console.log() to display JavaScript values in the debugger window:
Example
<!DOCTYPE html>
<html>
<body>
<h1>My First Web Page</h1>
<script>
a = 5;
b = 6;
c = a + b;
console.log(c);</script>
</body>
</html>
Try it Yourself »
Tip: Read more about the console.log() method in our JavaScript Console Reference.
<!DOCTYPE html>
<html>
<body>
<h1>My First Web Page</h1>
<script>
a = 5;
b = 6;
c = a + b;
console.log(c);</script>
</body>
</html>
Try it Yourself »
Tip: Read more about the console.log() method in our JavaScript Console Reference.
Setting Breakpoints
In the debugger window, you can set breakpoints in the JavaScript code.
At each breakpoint, JavaScript will stop executing, and let you examine JavaScript values.
After examining values, you can resume the execution of code (typically with a play button).
Major Browsers' Debugging Tools
Normally, you activate debugging in your browser with F12, and select "Console" in the debugger menu.
Otherwise follow these steps:
Chrome
- Open the browser.
- From the menu, select tools.
- From tools, choose developer tools.
- Finally, select Console.
Firefox Firebug
- Open the browser.
- Go to the web page:
http://www.getfirebug.com
- Follow the instructions how to:
install Firebug
http://www.getfirebug.com
install Firebug
Internet Explorer
- Open the browser.
- From the menu, select tools.
- From tools, choose developer tools.
- Finally, select Console.
Opera
- Open the browser.
- Go to the webpage:
http://dev.opera.com
- Follow the instructions how to:
add a Developer Console button to your toolbar.
http://dev.opera.com
add a Developer Console button to your toolbar.
Safari Firebug
- Open the browser.
- Go to the webpage:
http://safari-extensions.apple.com
- Follow the instructions how to:
install Firebug Lite.
http://safari-extensions.apple.com
install Firebug Lite.
Safari Develop Menu
- Go to Safari, Preferences, Advanced in the main menu.
- Check "Enable Show Develop menu in menu bar".
- When the new option "Develop" appears in the menu:
Choose "Show Error Console".
Choose "Show Error Console".
Did You Know?
Debugging is the process of testing, finding, and reducing bugs (errors) in computer programs.
The first known computer bug was a real bug (an insect) stuck in the electronics.
Debugging is the process of testing, finding, and reducing bugs (errors) in computer programs.
The first known computer bug was a real bug (an insect) stuck in the electronics.
The first known computer bug was a real bug (an insect) stuck in the electronics.
JavaScript Errors - Throw and Try to Catch
JavaScript try and catch
The try statement allows you to define a block fo code to be tested for errors while it is being executed.
The catch statement allows you to define a block of code to be executed, if an error occurs in the try block.
The JavaScript statement try and catch come in pairs.
JavaScript Throws Errors
when an error occurs, JavaScript will normally stop and generate an error message.
The technical term for this is: JavaScript will throw an exception (throw an error).
JavaScript will actually create an Error object with two properties: name and message.
The throw Statement
The throw statement allows you to create a custom error.
Technically you can throw an exception (throw an error).
The exception can be a JavaScript String, a Number, a Boolean or an Object:
hrow "Too big"; // throw a textthrow 500; // throw a number
If you use throw together with try and catch, you can control program flow and generate custom error messages.
Input Validation Example
This example examines input. If the value is wrong, an exception (err) is thrown.
The exception (err) is caught by the catch statement and a custom error message is displayed:
<!DOCTYPE html>
<html>
<body>
<p>Please input a number between 5 and 10:</p>
<input id="demo" type="text">
<button type="button" onclick="myFunction()">Test Input</button>
<p id="p01"></p>
<script>
function myFunction() {
var message, x;
message = document.getElementById("p01");
message.innerHTML = "";
x = document.getElementById("demo").value;
try {
if(x == "") throw "empty";
if(isNaN(x)) throw "not a number";
x = Number(x);
if(x < 5) throw "too low";
if(x > 10) throw "too high";
}
catch(err) {
message.innerHTML = "Input is " + err;
}
}</script>
</body>
</html>
Try it Yourself »HTML Validation
The code above is just an example.
Modern browsers will often use a combination of JavaScript and built-in HTML validation, using predefined validation rules defined in HTML attributes:
<input id="demo" type="number" min="5" max="10" step="1"
You can read more about forms validation in a later chapter of this tutorial.
The finally Statement
The finally statement lets you execute code, after try and catch, regardless of the result.
try {
Block of code to try}
catch(err) {
Block of code to handle errors}
finally {
Block of code to be executed regardless of the try / catch result}
Block of code to try}
catch(err) {
Block of code to handle errors}
finally {
Block of code to be executed regardless of the try / catch result}
The Error Object
JavaScript has a built in error object that provides error information when an error occurs.
The error object provides two useful properties: name and message.
Error Object Properties
Property | Description |
---|---|
name | Sets or returns an error name |
message | Sets or returns an error message (a string) |
Error Name Values
Six different values can be returned by the error name property:
Error Name | Description |
---|---|
EvalError | An error has occurred in the eval() function |
RangeError | A number "out of range" has occurred |
ReferenceError | An illegal reference has occurred |
SyntaxError | A syntax error has occurred |
TypeError | A type error has occurred |
URIError | An error in encodeURI() has occurred |
The six different values are described below.
Eval Error
An EvalError indicates an error in the eval() function.
Newer versions of JavaScript does not throw any EvalError. Use SyntaxError instead.
Range Error
A RangeError is thrown if you use a number that is outside the range of legal values.
For example: You cannot set the number of significant digits of a number to 500.
Example
var num = 1;
try {
num.toPrecision(500); // A number cannot have 500 significant digits}
catch(err) {
document.getElementById("demo").innerHTML = err.name;
}
Try it Yourself »Non-Standard Error Object Properties
Mozilla and Microsoft defines some non-standard error object properties:
fileName (Mozilla)
lineNumber (Mozilla)
columnNumber (Mozilla)
stack (Mozilla)
description (Microsoft)
number (Microsoft)
lineNumber (Mozilla)
columnNumber (Mozilla)
stack (Mozilla)
description (Microsoft)
number (Microsoft)
Do not use these properties in public web sites. They will not work in all browsers.
Subscribe to:
Posts (Atom)